A Comprehensive Guide to Angular Data Binding
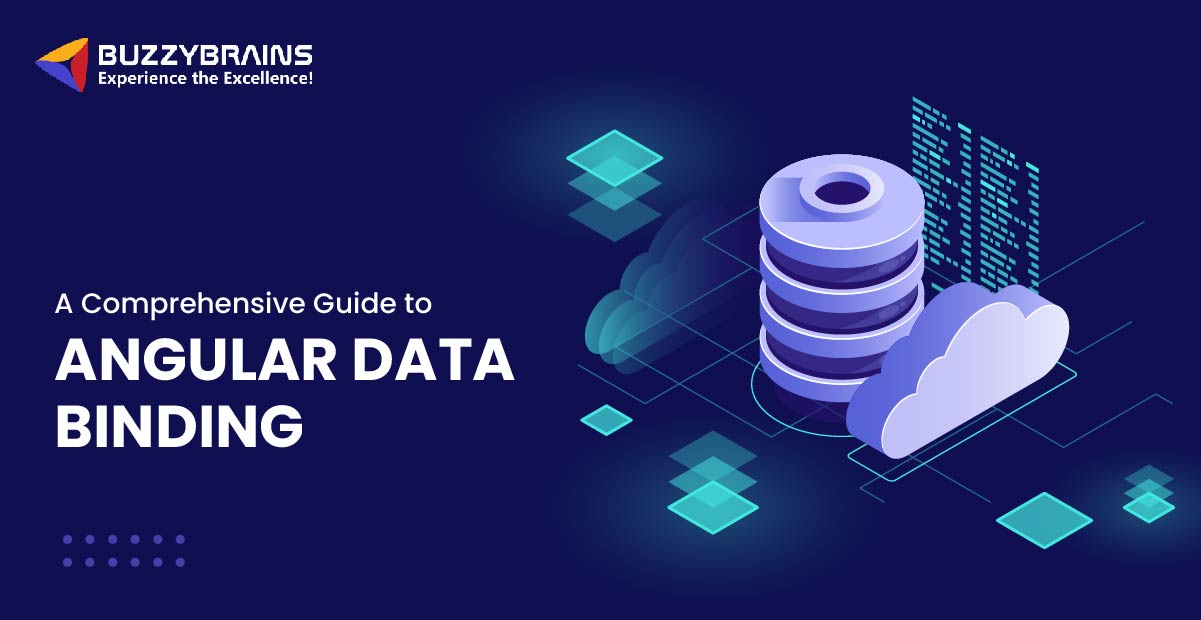
Angular has firmly established itself as one of the most popular frameworks for building dynamic web applications. According to recent statistics in 2024, Angular is used by over 17.1% of web developers worldwide, and the framework powers popular platforms like Google Cloud Console and Forbes. One of the key features that makes Angular so appealing is its powerful data binding capabilities, which streamline how developers manage communication between the user interface (UI) and the component logic.
As of 2023, Angular ranked among the top three JavaScript frameworks, with more than 1.7 million active websites utilizing its capabilities. A deep understanding of Angular’s data binding mechanism is essential for developers aiming to build responsive, efficient applications.
- What is Data Binding in Angular?
- Types of Angular Data Binding
- Benefits of Angular Data Binding
- How Angular Data Binding Works?
- Best Practices and Tips for Angular Data Binding
- Common Errors and Debugging Tips in Data Binding
- Advanced Concepts in Data Binding
- Real-World Applications of Angular Data Binding
- FAQs About Angular Data Binding
- Conclusion
- Streamline Development with Angular Expertise from BuzzyBrains!
What is Data Binding in Angular?
Data binding is the connection between a component’s logic and its corresponding user interface (UI) in Angular. This synchronization ensures that any changes in the application’s data are automatically reflected in the UI, and any updates made by the user in the UI are captured and passed back to the application logic.
In Angular, this dynamic interaction is made possible by various types of data binding mechanisms that allow you to manage the flow of data between your components and templates efficiently.
Types of Angular Data Binding
Data binding in Angular refers to the communication between the component class (the business logic) and the template (the view or UI). There are different types of data binding that allow developers to control how data flows between the model and the view. These can be categorized into one-way data binding and two-way data binding. Let’s dive deeper into each type.
1. One-Way Data Binding
One-way data binding allows data to flow in only one direction—either from the component to the view or from the view to the component. It is further divided into three types:
a. Interpolation
Interpolation binds data from the component to the template by embedding the component’s property values into the view. It is typically used to display dynamic data within HTML tags. Interpolation is done using double curly braces ({{ }}), where an expression inside the braces is evaluated and inserted into the DOM.
Example:
<p>Welcome, {{ username }}!</p>
In this example, username is a property in the component class. When the component renders, the value of username will be dynamically displayed inside the paragraph tag.
b. Property Binding
Property binding is another form of one-way data binding where you bind the property of an HTML element (such as a DOM element’s attribute, class, or style) to a value in the component. This allows dynamic values to be assigned to DOM properties in the template. The syntax involves using square brackets ([ ]) around the property.
Example:
html
Copy code
<img [src]="imageUrl" alt="Image not found">
In this example, the value of the src attribute is dynamically bound to the imageUrl property in the component class.
c. Event Binding
Event binding enables the communication from the view to the component. It captures user interactions, such as clicks, keystrokes, or mouse movements, and sends them to the component so it can execute corresponding logic. The syntax for event binding involves parentheses (( )) around the event.
Example:
<button (click)="onClick()">Click Me</button>
In this case, when the button is clicked, the onClick() method defined in the component is triggered.
2. Two-Way Data Binding
Two-way data binding allows data to flow in both directions, meaning changes in the component are reflected in the view, and changes made by the user in the view are automatically updated in the component. This type of binding is most commonly used in forms, where users input data, and the model updates in real-time.
Angular provides a special directive ngModel for two-way data binding, which binds both the value and the event. The syntax for two-way binding uses a combination of square brackets and parentheses, [( )], commonly referred to as “banana in a box.”
Example:
<input [(ngModel)]="username" placeholder="Enter your name">
<p>Your name is {{ username }}</p>
Here, the ngModel binds the username property in the component to the input field. Any change made by the user to the input field will update username, and changes in username will also update the input field.
Key Differences Between One-Way and Two-Way Data Binding
- One-Way Data Binding: Data flows in only one direction—either from the component to the view (interpolation, property binding) or from the view to the component (event binding).
- Two-Way Data Binding: Data flows in both directions, meaning the view and the component are synchronized.
3. Attribute Binding
Attribute binding allows you to set attributes directly in the HTML element by binding them to the component’s properties. This is useful for applying dynamic attributes to HTML elements, particularly for custom attributes.
Example:
<button [attr.aria-label]="buttonLabel">Click Me</button>
In this example, the aria-label attribute is dynamically set to the value of buttonLabel in the component.
4. Class Binding
Class binding allows you to dynamically add or remove classes from an HTML element based on the state of a property in the component.
Example:
<div [class.active]="isActive">Active Div</div>
In this example, the class active will be applied to the div element if isActive in the component is true.
5. Style Binding
Style binding allows you to bind the inline styles of an HTML element to a component’s properties. This helps in dynamically adjusting the styles based on the component’s logic.
Example:
<div [style.background-color]="isActive ? 'green' : 'red'">Color Box</div>
Here, the background color of the div will be set to green if isActive is true, and red if it is false.
Benefits of Angular Data Binding
Data binding in Angular provides several benefits that make development easier and more efficient.
- Simplifies interaction between UI and logic: Data binding reduces the amount of boilerplate code needed to keep the UI and the model in sync.
- Enhances user experience: With real-time updates, users can see immediate feedback from their actions without needing to refresh the page.
- Promotes maintainability: Angular’s clear data flow structure makes it easier to maintain and scale applications.
- Supports responsive design: By dynamically updating the view in response to data changes, Angular helps developers build responsive and interactive applications.
- Reduces errors: Automatic synchronization reduces the likelihood of inconsistencies between the model and the view.
How Angular Data Binding Works?
Angular uses a sophisticated mechanism to manage data binding behind the scenes. Understanding this flow can help you debug and optimize your applications.
- Change Detection: Angular’s change detection system tracks any changes to component data and updates the DOM accordingly.
- Component Interaction: Data flows from the component’s class to the template (in the case of property binding or interpolation) or from the template to the component (in the case of event binding).
- Zones: Angular uses zones to manage asynchronous operations, ensuring that any changes in data are tracked and propagated to the UI.
- Model-View Synchronization: In two-way data binding, Angular constantly synchronizes the model and view, updating both whenever a change is detected.
Best Practices and Tips for Angular Data Binding
Implementing best practices for data binding ensures your application remains efficient and scalable.
- Limit two-way data binding: Use two-way data binding sparingly to avoid performance overhead, especially in large applications.
- Optimize change detection: Implement OnPush change detection strategy to limit unnecessary updates and improve performance.
- Use trackBy in ngFor loops: When using ngFor, leverage trackBy to improve rendering performance for large lists.
- Leverage event delegation: Bind events at higher levels in the DOM where possible, rather than binding events to each individual child element.
- Use proper lifecycle hooks: Use hooks like ngOnChanges and ngAfterViewInit to manage component state and ensure that binding operations are completed after view initialization.
Common Errors and Debugging Tips in Data Binding
Errors in data binding can be frustrating, but many common issues have straightforward fixes.
- ExpressionChangedAfterItHasBeenCheckedError: This occurs when you modify data after Angular has performed change detection. Fix this by ensuring data updates happen before the change detection cycle.
- Undefined or null reference errors: These errors often occur when data binding is attempted on uninitialized variables. Initialize variables before binding them to the template.
- Event binding errors: Ensure event names are correctly spelled and that parentheses are properly placed when binding to events.
- ngModel issues: If two-way data binding with ngModel isn’t working, ensure that you’ve imported the FormsModule in your Angular module.
- Change detection performance issues: Overuse of two-way data binding can lead to performance problems. Monitor and optimize your application by adjusting change detection strategies.
Advanced Concepts in Data Binding
Once you’ve mastered the basics of Angular data binding, there are a few advanced concepts you should explore.
- Template Reference Variables: These allow you to access a DOM element or Angular component instance directly in the template.
- Event Binding with $event: This allows you to capture event data and pass it to the component for more complex logic.
- Binding to Custom Events: You can define and bind to custom events in Angular components using @Output and EventEmitter.
- Safe Navigation Operator (?.): This operator is used to prevent Angular from throwing errors when attempting to bind to potentially null or undefined objects.
- Custom Two-Way Binding: For more complex scenarios, you can create custom two-way data binding by combining input and output properties.
Real-World Applications of Angular Data Binding
Angular data binding has practical applications in various scenarios.
- Dynamic Forms: Two-way data binding is commonly used in dynamic forms where user input needs to be captured and stored in the model.
- Interactive Dashboards: Property and event binding are used in interactive dashboards to allow real-time data updates and user interaction with charts and graphs.
- E-commerce Platforms: In e-commerce applications, Angular data binding enables seamless updates of product details, cart totals, and stock levels.
- Chat Applications: Event binding is vital in chat applications to listen for user actions like sending messages, while data binding keeps conversations updated in real-time.
FAQs About Angular Data Binding
Here are some frequently asked questions related to Angular data binding.
Q1. How do you debug data binding issues in Angular?
To debug data binding issues, use Angular’s built-in error messages and developer tools. Additionally, you can check the console for binding errors and use Angular’s DevTools to trace change detection cycles.
Q2. What is two-way data binding in Angular?
Two-way data binding in Angular allows synchronization between the model and the view, ensuring that changes made in the view are reflected in the model and vice versa. This is achieved with the [(ngModel)] syntax.
Q3. What are the performance considerations for data binding in Angular?
Performance issues in data binding often arise due to excessive use of two-way data binding or inefficient change detection cycles. To improve performance, use OnPush change detection, trackBy in ngFor loops, and limit two-way data binding where possible.
Q4. What are common errors encountered in Angular data binding?
Common errors include ExpressionChangedAfterItHasBeenCheckedError, undefined or null reference errors, event binding errors, and issues with two-way data binding. Proper initialization of variables and careful use of change detection strategies can help avoid these errors.
Q5. How does Angular’s DevTools help in tracking data binding problems?
Angular’s DevTools allow you to inspect change detection cycles and identify areas where data binding issues might arise. It provides insights into performance bottlenecks and can help you optimize your application’s data flow.
Conclusion
Understanding Angular data binding is essential for any developer working with Angular. It streamlines the interaction between the UI and the underlying logic, creating dynamic, user-friendly applications. By mastering data binding and implementing best practices, you can enhance the performance and maintainability of your Angular projects.
Streamline Development with Angular Expertise from BuzzyBrains!
At BuzzyBrains, we specialize in delivering Angular-based solutions tailored to your business needs. Whether you’re building a dynamic web application or enhancing an existing platform, our experts are here to help. Contact us today to learn how we can optimize your Angular projects for success!